Como converter facilmente String para Integer em JAVA
Existem duas maneiras de converter String para Integer em Java,
- String para Integer usando Integer.parseInt()
- String para Integer usando Integer.valueOf()
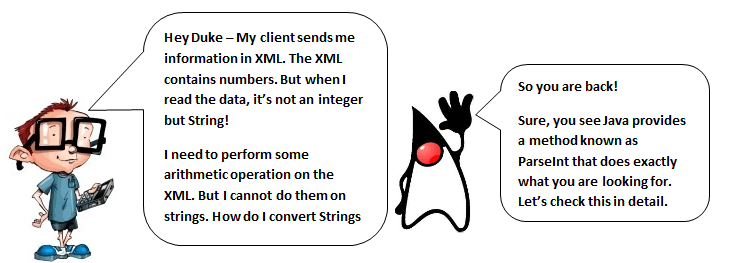
Digamos que você tenha uma string – strTest – que contém um valor numérico.
String strTest = “100”;
Tente realizar alguma operação aritmética como dividir por 4 – Isso mostra imediatamente um erro de compilação.
class StrConvert{ public static void main(String []args){ String strTest = "100"; System.out.println("Using String:" + (strTest/4)); } }
Saída:
/StrConvert.java:4: error: bad operand types for binary operator '/' System.out.println("Using String:" + (strTest/4));
Portanto, você precisa converter uma String em int antes de realizar operações numéricas nela
Exemplo 1:Converter String em Integer usando Integer.parseInt()
Sintaxe do método parseInt da seguinte forma:
int <IntVariableName> = Integer.parseInt(<StringVariableName>);
Passe a variável string como argumento.
Isso converterá o Java String para java Integer e o armazenará na variável inteira especificada.
Verifique o trecho de código abaixo-
class StrConvert{ public static void main(String []args){ String strTest = "100"; int iTest = Integer.parseInt(strTest); System.out.println("Actual String:"+ strTest); System.out.println("Converted to Int:" + iTest); //This will now show some arithmetic operation System.out.println("Arithmetic Operation on Int: " + (iTest/4)); } }
Saída:
Actual String:100 Converted to Int:100 Arithmetic Operation on Int: 25
Exemplo 2:Converter String em Integer usando Integer.valueOf()
O método Integer.valueOf() também é usado para converter String em Integer em Java.
A seguir, o exemplo de código mostra o processo de uso do método Integer.valueOf():
public class StrConvert{ public static void main(String []args){ String strTest = "100"; //Convert the String to Integer using Integer.valueOf int iTest = Integer.valueOf(strTest); System.out.println("Actual String:"+ strTest); System.out.println("Converted to Int:" + iTest); //This will now show some arithmetic operation System.out.println("Arithmetic Operation on Int:" + (iTest/4)); } }
Saída:
Actual String:100 Converted to Int:100 Arithmetic Operation on Int:25
NumberFormatException
NumberFormatException é lançado Se você tentar analisar uma seqüência de números inválida. Por exemplo, String 'Guru99' não pode ser convertido em Integer.
Exemplo:
public class StrConvert{ public static void main(String []args){ String strTest = "Guru99"; int iTest = Integer.valueOf(strTest); System.out.println("Actual String:"+ strTest); System.out.println("Converted to Int:" + iTest); } }
O exemplo acima fornece a seguinte exceção na saída:
Exception in thread "main" java.lang.NumberFormatException: For input string: "Guru99"
Java
- Como implantar aplicativos Java EE no Azure
- Strings Java
- Java enum Strings
- Como criar uma matriz de objetos em Java
- Método String Length () em Java:como encontrar com exemplo
- Método Java String charAt() com exemplo
- Método Java String compareTo():como usar com exemplos
- Java String EndsWith() Método com Exemplo
- Java String replace(), replaceAll() e método replaceFirst()
- Métodos Java String toLowercase() e toUpperCase()