Jogo Arduino Touch Breakout
Componentes e suprimentos
![]() |
| × | 1 | |||
| × | 1 |
Aplicativos e serviços online
![]() |
|
Sobre este projeto
Esta é uma versão pequena do clássico videogame de breakout para Arduino UNO e tela TFT LCD (240x320 pixels), driver ILI9341 com comunicação paralela de 8 bits.
O jogo
Este Breakout tem várias telas com diferentes linhas e colunas de tijolos configuráveis, até oito linhas, com cada duas linhas de uma cor diferente, que podem ser ativadas ou desativadas com padrões diferentes. Usando uma única bola, usando o painel de toque, o jogador deve derrubar tantos tijolos quanto possível, usando as paredes e / ou a raquete abaixo para ricochetear a bola contra os tijolos e eliminá-los. Se a raquete do jogador errar o rebote da bola, eles perderão uma vez.
Cada tijolo da linha ganha pontos diferentes cada.
uint8_t pointsForRow [] ={7, 7, 5, 5, 3, 3, 1, 1};
Cada nível pode configurar o tamanho da raquete e o tamanho da bola. A velocidade da bola aumenta a cada golpe, você pode configurar a velocidade inicial para cada tela. Dependendo do ponto da raquete que atinge a bola, a velocidade horizontal também muda
Você pode definir como novas telas com diferentes padrões de parede:
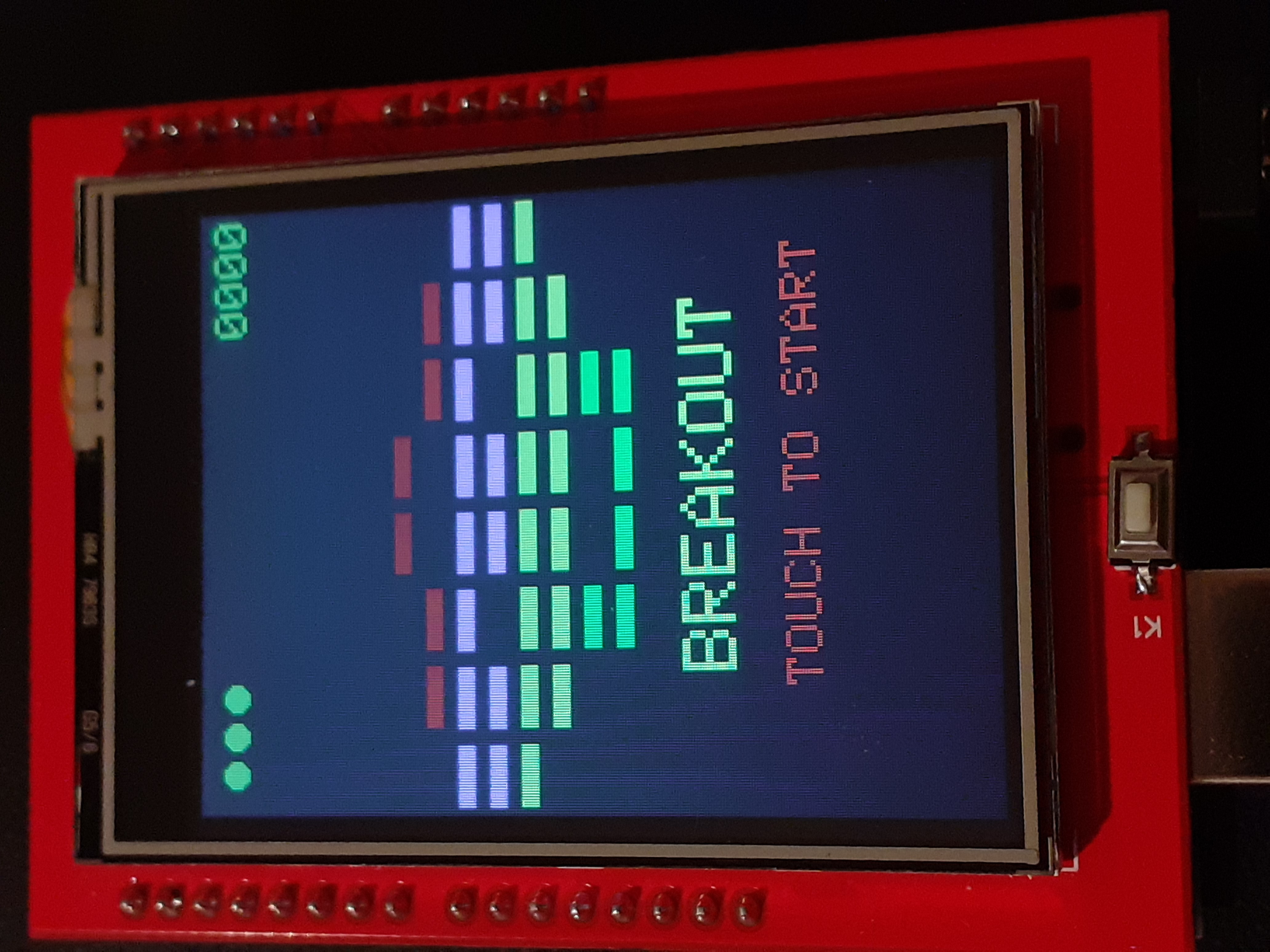
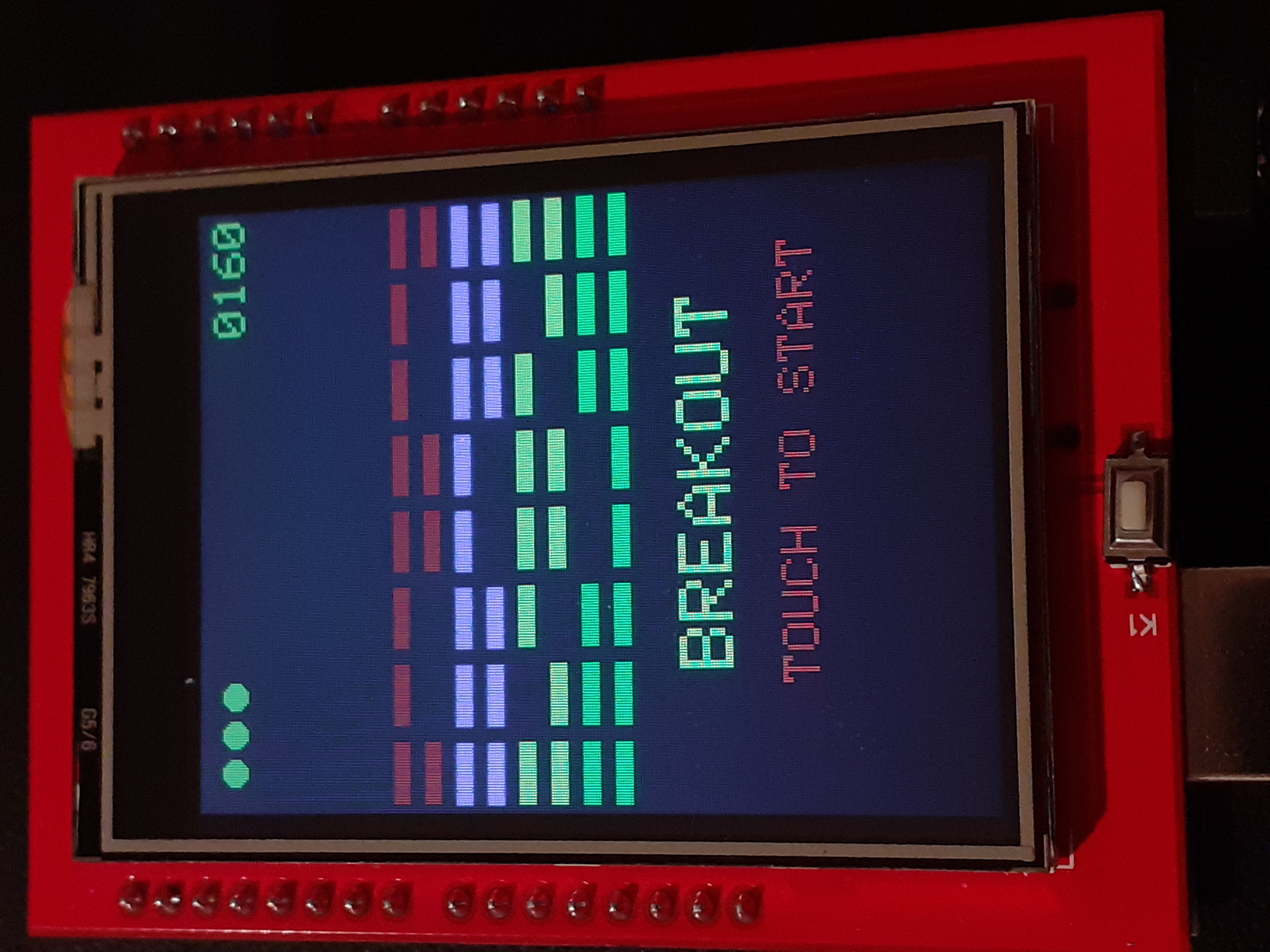
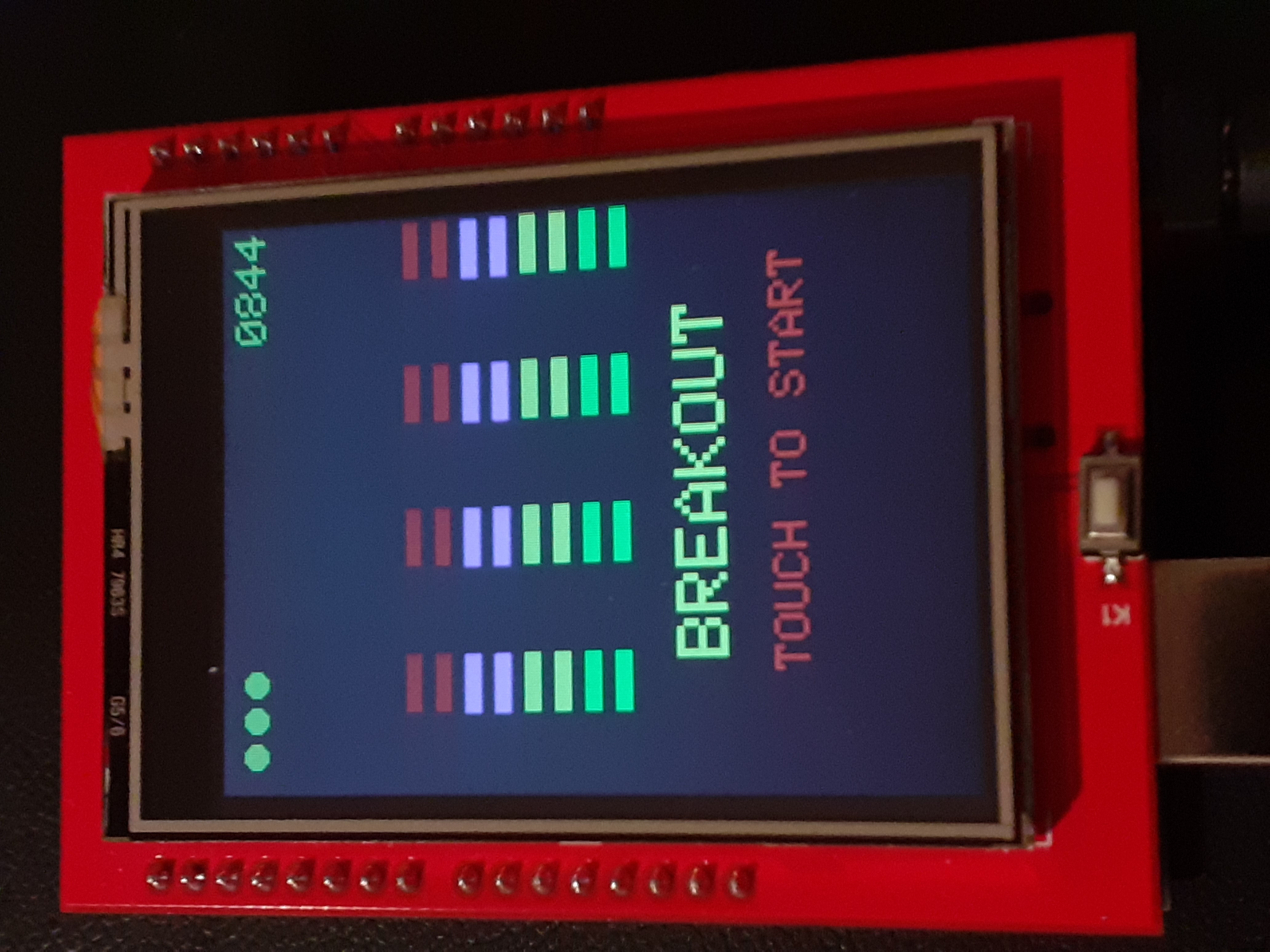
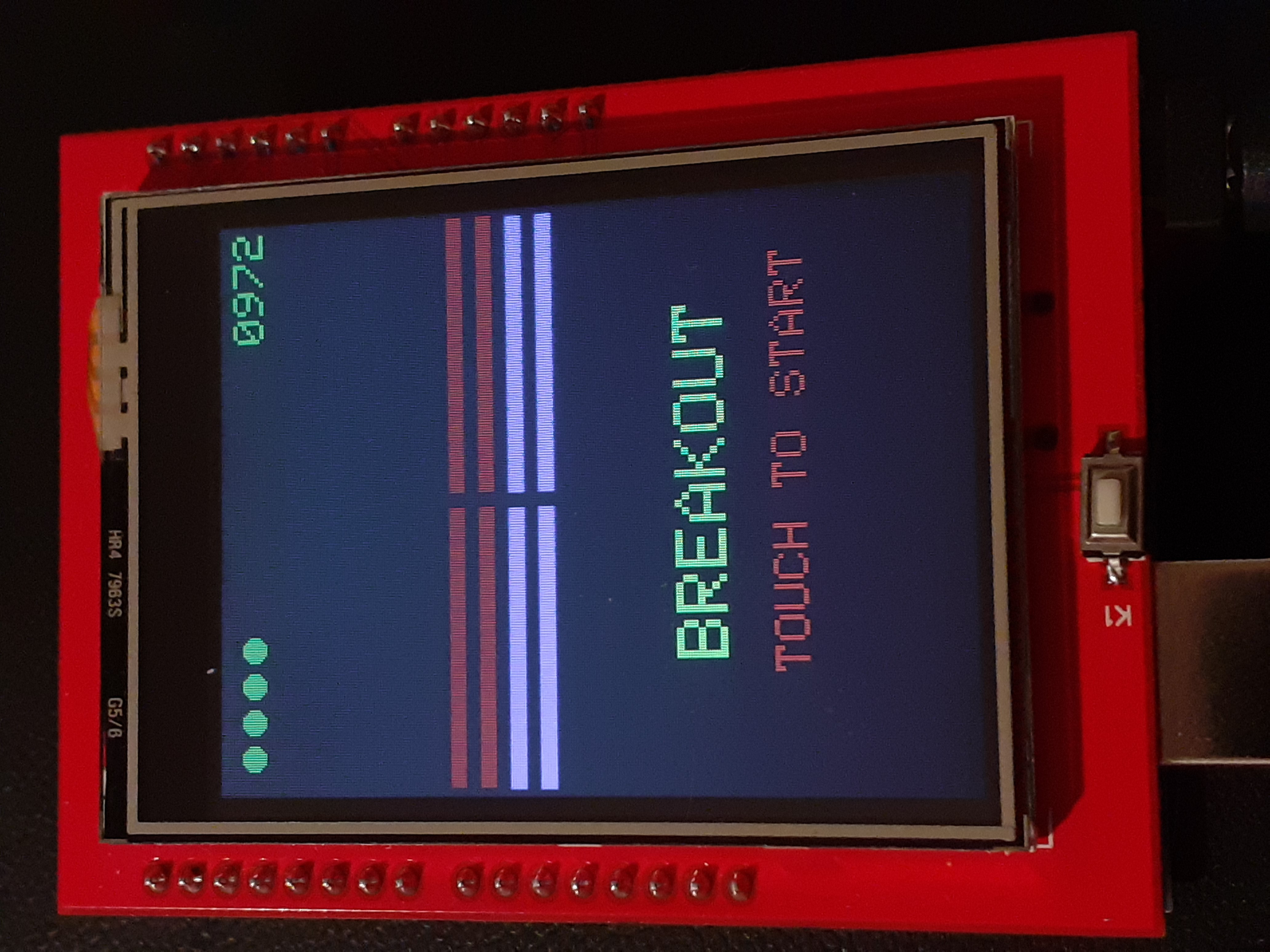
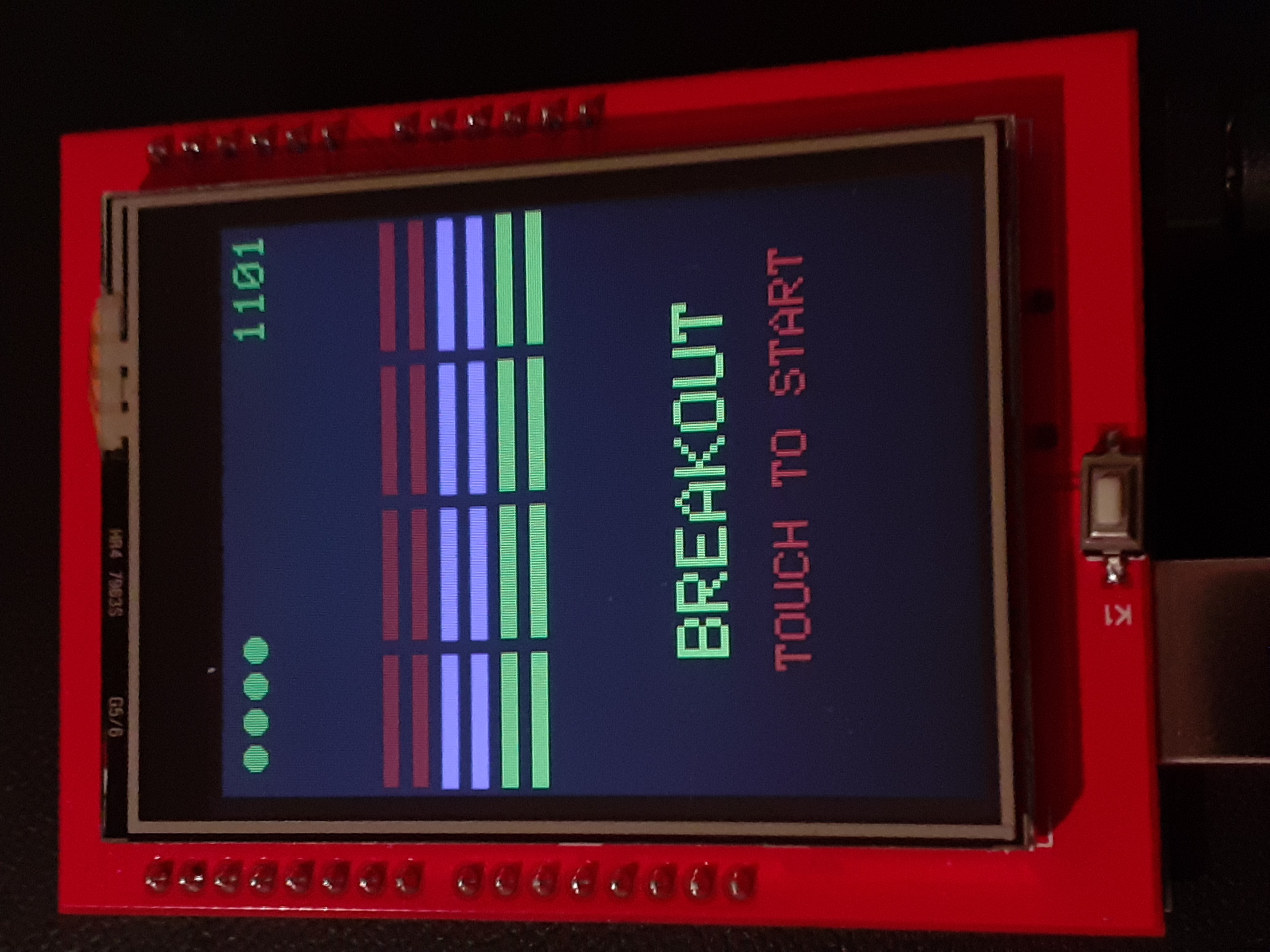
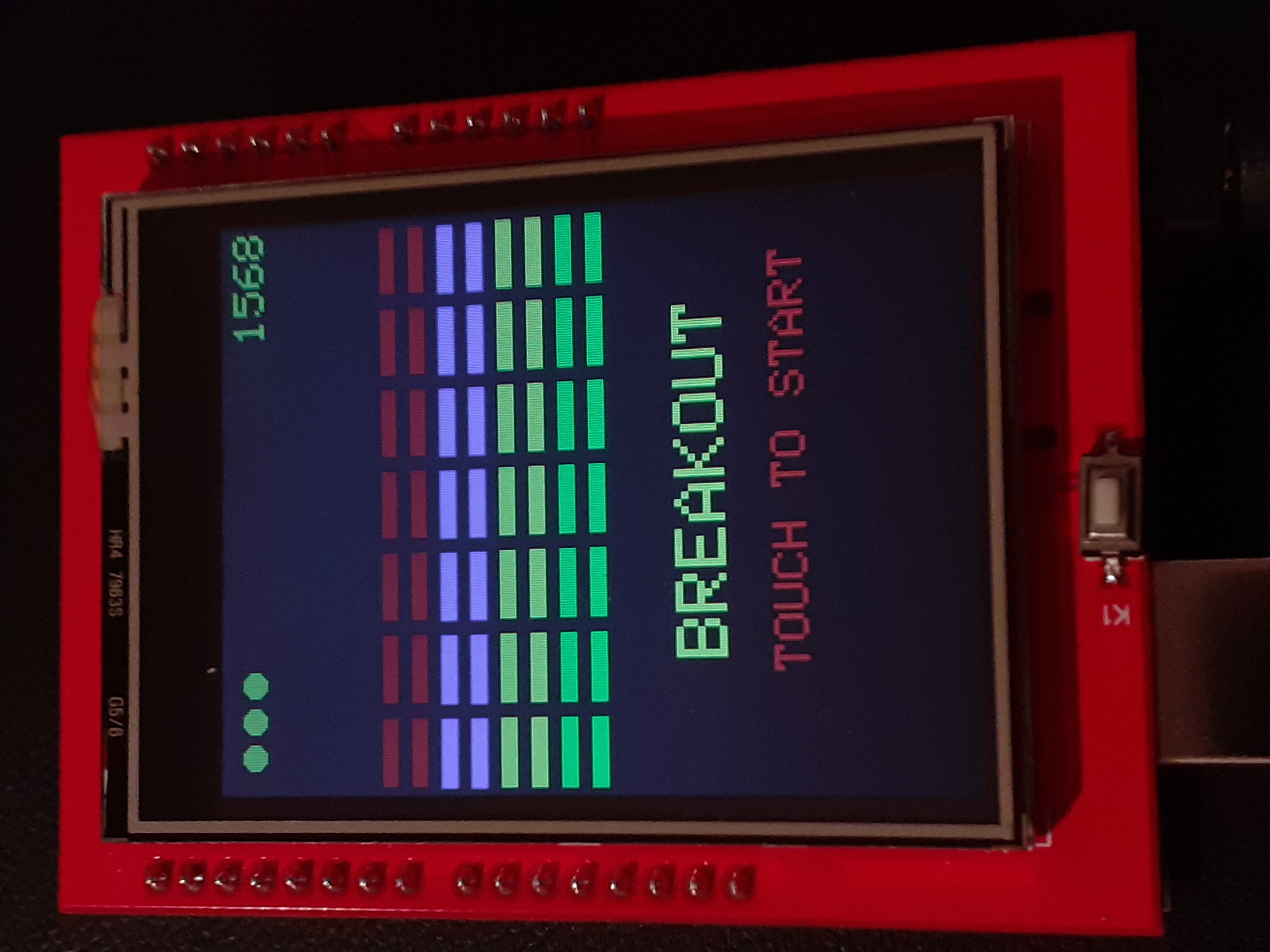
Como jogar
Segure o dispositivo com as mãos e use os dedos dos polegares sobre a tela para mover a raquete para a esquerda ou para a direita.
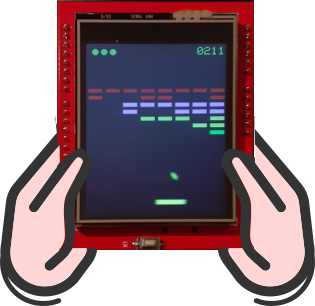
Defina uma nova tela
Esta estrutura é usada para definir uma nova tela:
typedef struct game_type {
int ballsize;
int playerwidth;
int playerheight;
int expoent;
int top;
int linhas;
colunas int;
int brickGap;
vidas int;
parede int [GAMES_NUMBER];
int initVelx;
int initVely;
} tipo_de_jogo;
e adicione a nova tela ao conjunto:
game_type games [GAMES_NUMBER] =
// ballsize, playerwidth, playerheight, expoente, topo, linhas, colunas, brickGap, vidas, parede [8], initVelx, initVely
{
{10, 60, 8, 6, 40, 8, 8, 3, 3, {0x18, 0x66, 0xFF, 0xDB, 0xFF, 0x7E, 0x24, 0x3C}, 28, -28},
Padrão de parede
O padrão de parede é definido como uma matriz de 8x8 bits
ej.
{0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA}
que corresponde a esta matriz de bits
1,0,1,0,1,0,1,0
1,0,1,0,1,0,1,0
1,0,1, 0,1,0,1,0
1,0,1,0,1,0,1,0
1,0,1,0,1,0,1,0
1,0,1,0,1,0,1,0
1,0,1,0,1,0,1,0
1,0,1,0,1,0 , 1,0
Irá produzir esta parede, observe que ela é espelhada:
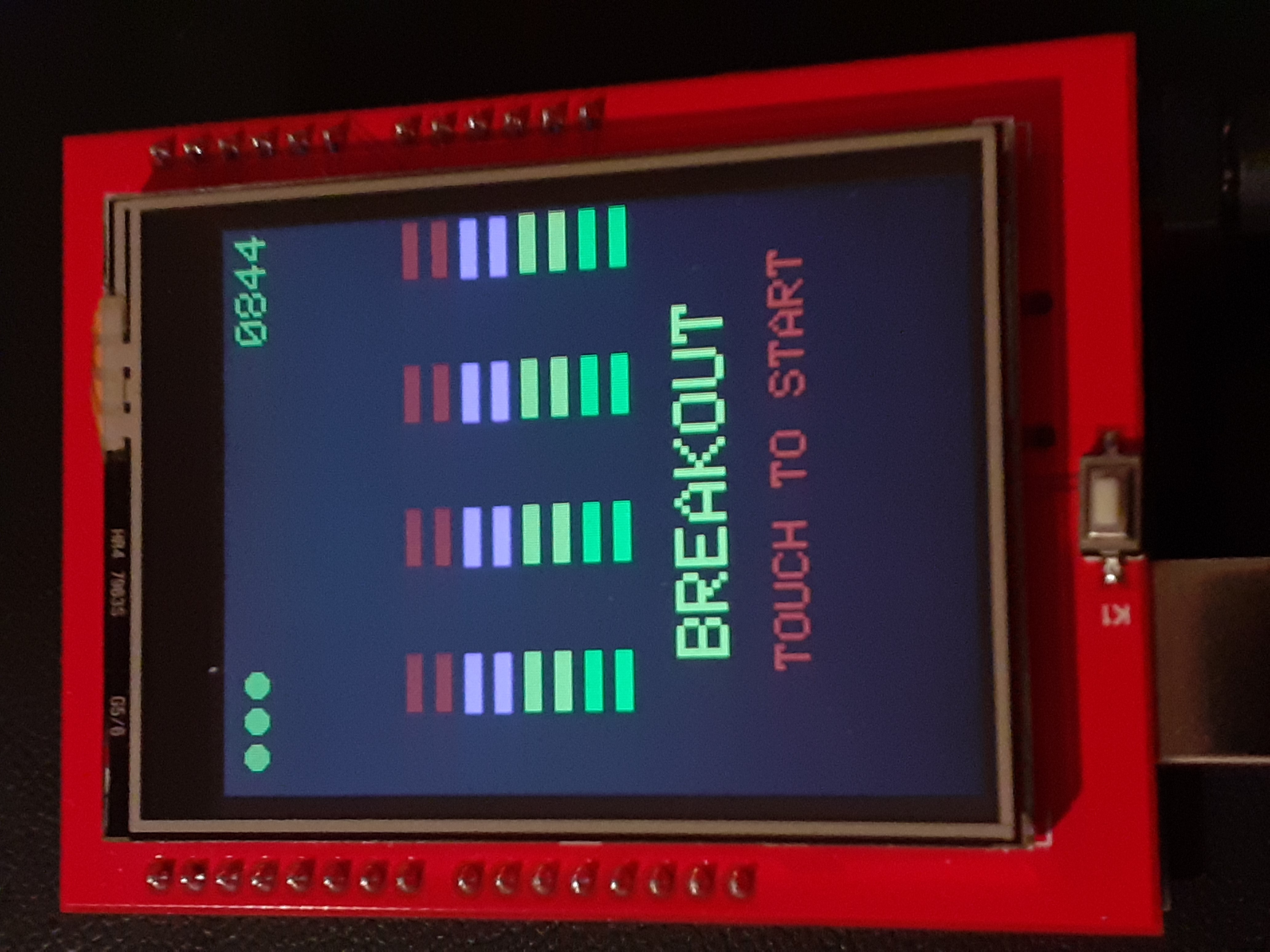
Hardware
Este projeto usa um display AZ-Delivery TFT LCD de 2,4 polegadas com touchscreen resistiva de 4 fios e um leitor de cartão SD integrado. Tela LCD TFT de 2,4 polegadas AZ-Delivery.
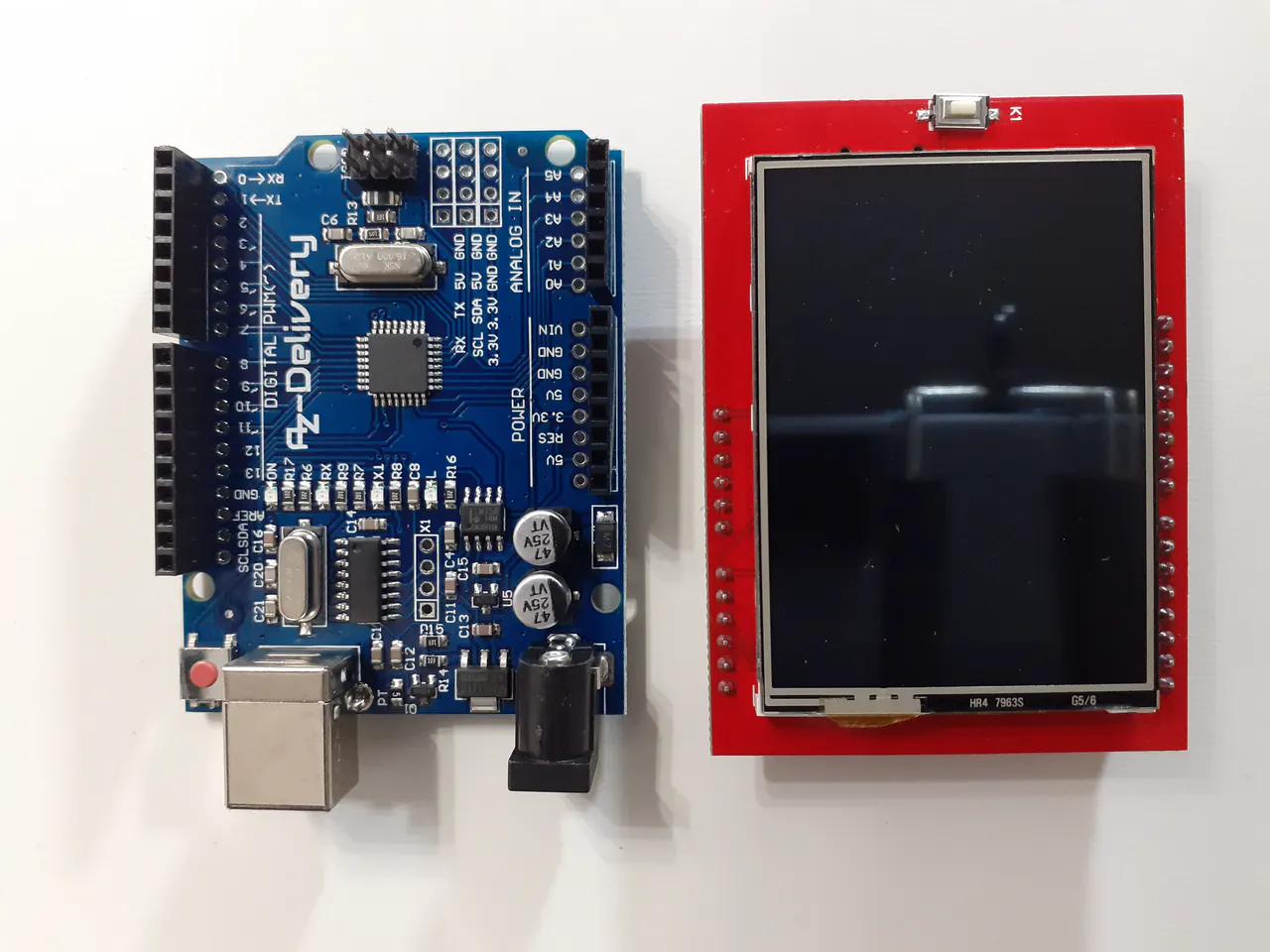
Mais sobre este escudo em meu artigo "Testando em estrada um AZ-Delivery 2, 4 TFT LCD Touch Display"
Montagem do escudo
Você só precisa conectar o escudo sobre o Aduino.
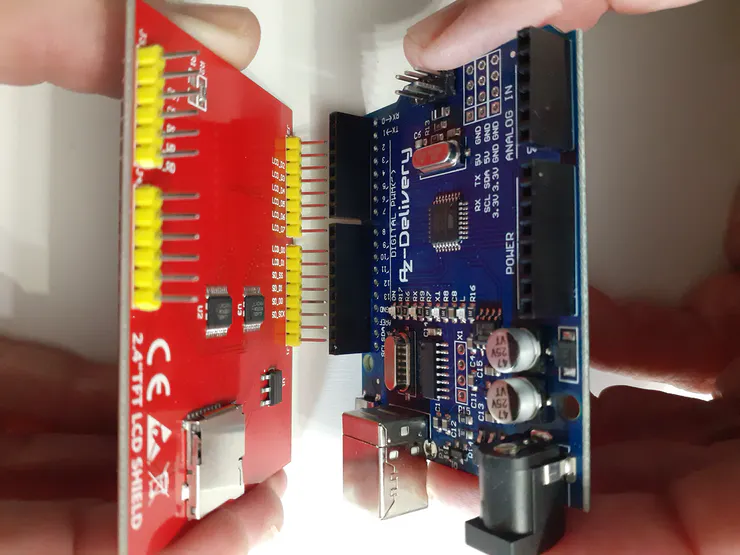
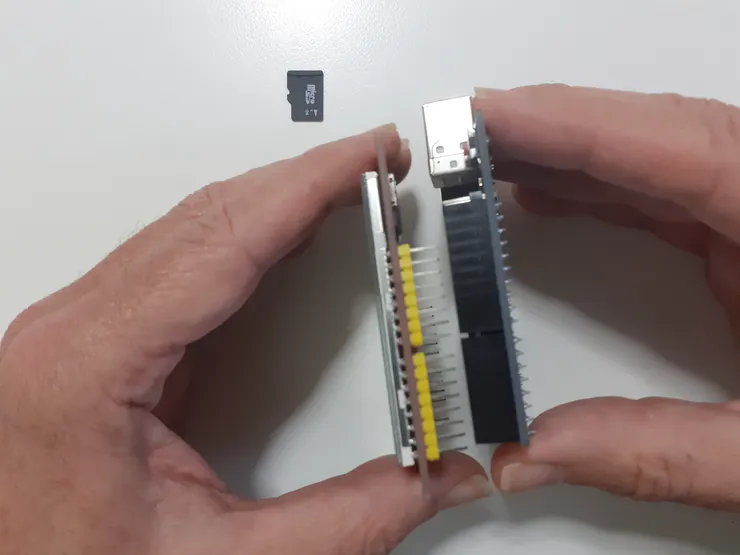
Bibliotecas
#include // Biblioteca gráfica principal
#include
#include
Calibrando a tela de toque
Você deve calibrar a tela para que as informações de posição estejam corretas ao tocar na tela. A biblioteca MCUFriend_kbv fornece um exemplo com o nome "TouchScreen_Calibr_native". O exemplo envia os resultados para a porta serial. Inicie o monitor serial do Arduino IDE para que você possa copiar o código gerado pelo exemplo.
Siga as instruções na tela de toque, pressione e segure os marcadores de posição exibidos, que são destacados em branco. Depois de fazer todas as marcas de posição, a calibração da tela é enviada para você na tela de toque e pela porta serial.
Para este projeto, você precisa dos dados para a "calibração retrato".
TouchScreen.h GFX Calibration
Fazendo todos os pinos de controle e barramento INPUT_PULLUP
O pullup analógico típico de 30k com o pino correspondente
teria leitura baixa quando o digital fosse escrito BAIXO
por ex. lê ~ 25 para 300R X direção
por ex. lê ~ 30 para 500R direção Y
Teste:(A2, D8) =26
Teste:(A3, D9) =28
ID =0x9341
cx =153 cy =103 cz =534 X, Y, Pressão
cx =150 cy =475 cz =406 X, Y, Pressão
cx =155 cy =868 cz =231 X, Y, Pressão
cx =517 cy =103 cz =561 X, Y, Pressão
cx =535 cy =855 cz =364 X, Y, Pressão
cx =884 cy =88 cz =650 X, Y, Pressão
cx =908 cy =478 cz =557 X, Y, Pressão
cx =902 cy =864 cz =488 X, Y, Pressão
*** COPY-PASTE do Terminal Serial:
const int XP =8, XM =A2, YP =A3, YM =9; // 240x320 ID =0x9341
const int TS_LEFT =118, TS_RT =931, TS_TOP =72, TS_BOT =887;
CALIBRAÇÃO DE RETRATO 240 x 320
x =mapa (px, LEFT =118 , RT =931, 0, 240)
y =mapa (py, TOP =72, BOT =887, 0, 320)
CALIBRAÇÃO DE PAISAGEM 320 x 240
x =mapa (py, LEFT =72, RT =887, 0, 320)
y =mapa (px, TOP =931, BOT =118, 0, 240)
Animação
Para mover a imagem pela tela ao longo do tempo, é preciso usar uma velocidade estática e aplicá-la à posição de uma imagem a cada intervalo de tempo.
pos + =vel * dt;
Evitando aritmética de ponto flutuante
A resolução do ILI9341 é 240 x 320, portanto, precisamos de dois inteiros de 9 bits para fazer referência a um pixel na tela. Usando inteiros de 16 bits, deixa 6 bits livres para representar uma parte decimal.
nnnn nnnn nndd dddd
Chamamos esse número, 6, de expoente binário. E podemos usar esses seis bits para ter uma parte decimal variando de 0,000 a 0,63. Portanto, podemos usar matemática inteira evitando aritmética de ponto flutuante.
Para obter a parte inteira do número, fazemos um deslocamento aritmético para a direita.
número>> expoente
state.ballx + =state.velx;
state.bally + =state.vely;
// verifica as colisões de bolas e sai
checkBallCollisions (jogo , &estado, estado.ballx>> jogo-> expoente, estado.bally>> jogo-> expoente);
checkBallExit (jogo, &estado, estado.ballx>> jogo-> expoente, estado.bally>> jogo -> expoente);
Modo de demonstração
Remova o comentário da Diretriz de Definição e o remo seguirá a bola, como pode ser visto nos vídeos de demonstração:
#define DEMO_MODE
Aproveite!
Crie novos padrões e níveis e compartilhe-os!
Código
- Arduino Breakout
Arduino Breakout Arduino
/ * Arduino Touch TFT Breakout Jogo de breakout clássico Peças necessárias:Ardunio UNO AZ-Delivery 2.4 Tela LCD TFT Touch Arduino Shield ou compatível Este código de exemplo é de domínio público. Modificado em 07 11 2020 por Enrique Albertos * /// #define DEMO_MODE # include// Biblioteca de gráficos principais # include #include #define BLACK 0x0000 # define BLUE 0x001F # define RED 0xF800 # define GREEN 0x07E0 # define CYAN 0x07FF # define MAGENTA 0xF81F # define YELLOW 0xFFE0 # define WHITE 0xFFFF # define PRIMARY_COLOR 0x4A11 # define PRIMARY_LIGHT_COLOR 0x7A17 # define PRIMARY_DARK_COLOR 0x4016 # define o chip PRIMARY_COLOR 0x4A11 # define PRIMARY_COLOR 0x7A17 # define PRIMARY_DARK_COLOR 0x4016 # define PRIMARY_TEXT_COLOR A3 0x4016 # define o chip Analógico # 0x_COLT 0x_COLOR 3 //_COLOR vai para o Chip Analógico A3 0x_COLOR 3 // Define o chip LCD F3 0x_COLOR3 //_COLOR vai para Analógico # 0x_COLT. #define LCD_CD A2 // Comando / Dados vai para Analógico 2 # define LCD_WR A1 // Gravação de LCD vai para Analógico 1 # define LCD_RD A0 // Leitura de LCD vai para Analógico 0 # define LCD_RESET A4 // Pode alternadamente conectar ao reset do Arduino pinAdafruit_TFTLCD tft (LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET); # define LOWFLASH (definido (__ AVR_ATmega328P__) &&defined (MCUFRIEND_KBV_H _)) // Pressão da tela sensível ao toque # define MINPRESSURE 40 # int_tprESSA calibragem MAX16 toque MAX16 Limite da tela =1000 toque_t_t16_t16 configuração MAX16 8, XM =A2, YP =A3, YM =9; // 240x320 ID =0x9341const int16_t TS_LEFT =122, TS_RT =929, TS_TOP =77, TS_BOT =884; const TouchScreen ts =TouchScreen (XP, YP, XM, YM, 300); # define SCORE_SIZE 30char scoreFormat [] ="% 04d "; typedef struct gameSize_type {int16_t x, y, width, height;} gameSize_type; gameSize_type gameSize; uint16_t backgroundColor =BLACK; nível interno; const uint8_t BIT_MASK [] ={0x01, 0x02, 0x4004, 0x08, 0x10, 0x20, 0x4004, 0x08, 0x10, 0x20, 0x4004, 0x08, 0x10, 0x20, 0x4004 , 0x80}; uint8_t pointsForRow [] ={7, 7, 5, 5, 3, 3, 1, 1}; # define GAMES_NUMBER 16typedef struct game_type {int ballsize; int playerwidth; int playerheight; expoente int; int top; linhas internas; colunas int; int brickGap; vidas int; parede interna [GAMES_NUMBER]; int initVelx; int initVely;} game_type; game_type games [GAMES_NUMBER] =// ballize, playerwidth, playerheight, expoente, topo, linhas, colunas, brickGap, vidas, parede [8], initVelx, initVely {{10, 60, 8, 6, 40, 8, 8, 3, 3, {0x18, 0x66, 0xFF, 0xDB, 0xFF, 0x7E, 0x24, 0x3C}, 28, -28}, {10, 50, 8, 6, 40, 8, 8, 3 , 3, {0xFF, 0x99, 0xFF, 0xE7, 0xBD, 0xDB, 0xE7, 0xFF}, 28, -28}, {10, 50, 8, 6, 40, 8, 8, 3, 3, {0xAA, 0x55 , 0xAA, 0x55, 0xAA, 0x55, 0xAA, 0x55}, 28, -28}, {8, 50, 8, 6, 40, 8, 8, 3, 3, {0xFF, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xFF}, 34, -34}, {10, 40, 8, 6, 40, 8, 8, 3, 3, {0xFF, 0xAA, 0xAA, 0xFF, 0xFF, 0xAA, 0xAA, 0xFF}, 28, -28}, {10, 40, 8, 6, 40, 8, 8, 3, 3, {0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA}, 28, -28}, { 12, 64, 8, 6, 60, 4, 2, 3, 4, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 20, -20}, {12, 60, 8, 6 , 60, 5, 3, 3, 4, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 22, -22}, {10, 56, 8, 6, 30, 6, 4, 3, 4, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 24, -24}, {10, 52, 8, 6, 30, 7, 5, 3, 4, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 26, -26 }, {8, 48, 8, 6, 30, 8, 6, 3, 3, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 28, -28}, {8, 44, 8, 6, 30, 8, 7, 3, 3, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 30, -30}, {8, 40, 8, 6, 30, 8 , 8, 3, 3, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 32, -32}, {8, 36, 8, 6, 40, 8, 8, 3, 3, {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}, 34, -34}, {8, 36, 8, 6, 40, 8, 8, 3, 3, {0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA, 0xAA}, 34, -34}}; game_type * game; typedef struct game_state_type {uint16_t ballx; uint16_t bally; uint16_t ballxold; uint16_t ballyold; int velx; int vamente; int playerx; int playerxold; int wallState [8]; pontuação int; int restanteLives; int top; int bottom; int walltop; int wallbottom; int brickheight; int brickwidth;}; game_state_type state; ////////////////////////////////////////////// ////////////////////////// ARDUINO SETUP ////////////////////////////// //////////////////////////////////////// void setup () {initTft (tft); gameSize ={0, 0, tft.width (), tft.height ()}; newGame (&games [0], &state, tft);} ///////////////////////////////////////// /////////////////////////////// ARDUINO LOOP /////////////////////// ///////////////////////////////////////////// Int selection =-1; void loop ( void) {selection =readUiSelection (jogo, &estado, seleção); drawPlayer (jogo e estado); // armazena a posição antiga para remover pixels antigos state.playerxold =state.playerx; // calcular a nova posição da bola x1 =x0 + vx * dt // verificar a velocidade máxima if (abs (state.vely)> ((1 < expoent) - 1)) {state.vely =((1 < expoente) - 1) * ((state.vely> 0) - (state.vely <0)); } if (abs (state.velx)> ((1 < expoente) - 1)) {state.velx =((1 < expoente) - 1) * ((state.velx> 0 ) - (state.velx <0)); } state.ballx + =state.velx; state.bally + =state.vely; // verifica as colisões de bola e sai checkBallCollisions (game, &state, state.ballx>> game-> expoent, state.bally>> game-> expoent); checkBallExit (jogo, &estado, estado.ballx>> jogo-> expoente, estado.bally>> jogo-> expoente); // desenha a bola na nova posição drawBall (state.ballx>> game-> expoent, state.bally>> game-> expoent, state.ballxold>> game-> expoent, state.ballyold>> game-> expoente, game -> tamanho da bola); // armazena a posição antiga para remover pixels antigos state.ballxold =state.ballx; state.ballyold =state.bally; // incrementa a velocidade state.velx =(20 + (state.score>> 3)) * ((state.velx> 0) - (state.velx <0)); state.vely =(20 + (state.score>> 3)) * ((state.vely> 0) - (state.vely <0)); // se nenhum tijolo vai para o próximo nível if (noBricks (jogo, &estado) &&nível vidas, estado-> restantes vidas); updateScore (estado-> pontuação); setupWall (jogo, estado); touchToStart (); clearDialog (gameSize); updateLives (jogo-> vidas, estado-> restantes vidas); updateScore (estado-> pontuação); setupWall (game, state);} void setupStateSizes (game_type * game, game_state_type * state, Adafruit_TFTLCD &tft) {state-> bottom =tft.height () - 30; estado-> brickwidth =tft.width () / jogo-> colunas; estado-> brickheight =tft.height () / 24;} void setupState (game_type * game, game_state_type * state, Adafruit_TFTLCD &tft) {setupStateSizes (game, state, tft); para (int i =0; i linhas; i ++) {estado-> wallState [i] =0; } estado-> playerx =tft.width () / 2 - jogo-> playerwidth / 2; estado-> restantes vidas =jogo-> vidas; estado-> bally =estado-> inferior < expoente; estado-> ballyold =estado-> inferior < expoente; estado-> velx =jogo-> initVelx; state-> vely =game-> initVely;} void updateLives (int vidas, int restanteLives) {para (int i =0; i walltop =game-> top + 40; state-> wallbottom =state-> walltop + game-> rows * state-> brickheight; para (int i =0; i linhas; i ++) {for (int j =0; j colunas; j ++) {if (isBrickIn (jogo-> parede, j, i)) {setBrick (estado-> wallState, j, i); drawBrick (estado, j, i, cores [i]); }}}} void drawBrick (game_state_type * state, int xBrick, int yBrickRow, uint16_t backgroundColor) {tft.fillRect ((state-> brickwidth * xBrick) + game-> brickGap, state-> walltop + (state-> brickheight * yBrickRow) + game-> brickGap, estado-> brickwidth - game-> brickGap * 2, state-> brickheight - game-> brickGap * 2, backgroundColor);} boolean noBricks (game_type * game, game_state_type * state) {for ( int i =0; i linhas; i ++) {if (estado-> wallState [i]) retorna falso; } return true;} void drawPlayer (game_type * game, game_state_type * state) {// pintar tft.fillRect (state-> playerx, state-> bottom, game-> playerwidth, game-> playerheight, YELLOW); if (state-> playerx! =state-> playerxold) {// remove pixels antigos if (state-> playerx playerxold) {tft.fillRect (state-> playerx + game-> playerwidth, state-> bottom , abs (estado-> playerx - estado-> playerxold), jogo-> playerheight, backgroundColor); } else {tft.fillRect (estado-> playerxold, estado-> bottom, abs (estado-> playerx - estado-> playerxold), game-> playerheight, backgroundColor); }}} void drawBall (int x, int y, int xold, int yold, int ballsize) {// remover pixels antigos // if (xold! =x &&yold! =y) {if (xold <=x &&yold <=y) {tft.fillRect (xold, yold, ballsize, y - yold, BLACK); tft.fillRect (xold, yold, x - xold, ballsize, BLACK); } else if (xold> =x &&yold> =y) {tft.fillRect (x + tamanho da bola, yold, xold - x, tamanho da bola, PRETO); tft.fillRect (xold, y + ballsize, ballsize, yold - y, BLACK); } else if (xold <=x &&yold> =y) {tft.fillRect (xold, yold, x - xold, ballsize, BLACK); tft.fillRect (xold, y + ballsize, ballsize, yold - y, BLACK); } else if (xold> =x &&yold <=y) {tft.fillRect (xold, yold, ballsize, y - yold, BLACK); tft.fillRect (x + tamanho da bola, yold, xold - x, tamanho da bola, PRETO); } // pinta uma nova bola tft.fillRect (x, y, ballsize, ballsize, YELLOW); //}} void touchToStart () {drawBoxedString (0, 200, "BREAKOUT", 3, AMARELO, PRETO); drawBoxedString (0, 240, "TOUCH TO START", 2, RED, BLACK); while (waitForTouch () <0) {}} void gameOverTouchToStart () {drawBoxedString (0, 180, "GAME OVER", 3, AMARELO, PRETO); drawBoxedString (0, 220, "TOUCH TO START", 2, RED, BLACK); while (waitForTouch () <0) {}} void updateScore (int score) {char buffer [5]; snprintf (buffer, sizeof (buffer), scoreFormat, score); drawBoxedString (tft.width () - 50, 6, buffer, 2, AMARELO, PRIMARY_DARK_COLOR);} void checkBrickCollision (game_type * jogo, game_state_type * estado, uint16_t x, uint16_t y) {int x1 =x + game-> ballsize; int y1 =y + jogo-> tamanho da bola; colisões internas =0; colisões + =checkCornerCollision (jogo, estado, x, y); colisões + =checkCornerCollision (jogo, estado, x1, y1); colisões + =checkCornerCollision (jogo, estado, x, y1); colisões + =checkCornerCollision (jogo, estado, x1, y); if (colisões> 0) {state-> vely =(-1 * state-> vely); if ((((x% state-> brickwidth) ==0) &&(state-> velx <0)) || ((((x + game-> ballsize)% state-> brickwidth) ==0) &&(estado-> velx> 0))) {estado-> velx =(-1 * estado-> velx); }}} int checkCornerCollision (game_type * game, game_state_type * state, uint16_t x, uint16_t y) {if ((y> state-> walltop) &&(y wallbottom)) {int yBrickRow =(y - state-> walltop) / state-> brickheight; int xBrickColumn =(x / estado-> largura do bloco); if (isBrickIn (estado-> wallState, xBrickColumn, yBrickRow)) {hitBrick (state, xBrickColumn, yBrickRow); return 1; }} return 0;} void hitBrick (game_state_type * state, int xBrick, int yBrickRow) {state-> score + =pointsForRow [yBrickRow]; drawBrick (estado, xBrick, yBrickRow, WHITE); atraso (16); drawBrick (estado, xBrick, yBrickRow, BLUE); atraso (8); drawBrick (state, xBrick, yBrickRow, backgroundColor); unsetBrick (estado-> wallState, xBrick, yBrickRow); updateScore (state-> score);} void checkBorderCollision (game_type * game, game_state_type * state, uint16_t x, uint16_t y) {// verifique a colisão da parede if (x + game-> ballsize> =tft.width ()) {state -> velx =-abs (estado-> velx); } if (x <=0) {estado-> velx =abs (estado-> velx); } if (y <=SCORE_SIZE) {state-> vely =abs (state-> vely); } if (((y + jogo-> tamanho da bola)> =estado-> fundo) &&((y + jogo-> tamanho da bola) <=(estado-> fundo + jogo-> altura do jogador)) &&(x> =estado-> playerx) &&(x <=(estado-> playerx + game-> playerwidth))) {// alterar vel x próximo às fronteiras do jogador if (x> (state-> playerx + game-> playerwidth - 6)) {state -> velx =estado-> velx - 1; } else if (x playerx + 6) {estado-> velx =estado-> velx + 1; } estadual-> vely =-abs (estadual-> vely); }} void checkBallCollisions (game_type * game, game_state_type * state, uint16_t x, uint16_t y) {checkBrickCollision (game, state, x, y); checkBorderCollision (game, state, x, y);} void checkBallExit (game_type * game, game_state_type * estado, uint16_t x, uint16_t y) {if (((y + game-> ballsize)> =tft.height ())) {estado-> vidas restantes--; updateLives (jogo-> vidas, estado-> restantes vidas); atraso (500); estadual-> vely =-abs (estadual-> vely); }} void setBrick (int wall [], uint8_t x, uint8_t y) {wall [y] =wall [y] | BIT_MASK [x];} void unsetBrick (int wall [], uint8_t x, uint8_t y) {wall [y] =wall [y] &~ BIT_MASK [x];} booleano isBrickIn (int wall [], uint8_t x, uint8_t y) {return wall [y] &BIT_MASK [x];} ///////////////////////////////////////// /////////////////////////////////// TFT SETUP ///////////////////// /////////////////////////////////////////////// void initTft (Adafruit_TFTLCD &tft) {tft.reset (); uint16_t ID =tft.readID (); tft.begin (ID); tft.setRotation (0);} //////////////////////////////////////////// //////////////////////// Métodos de pintura de tela ///////////////////////////// ////////////////////////////////////////// ** Imprimir um texto em forecolor sobre uma caixa preenchida com cor de fundo. O tamanho do retângulo é calculado para incluir todo o texto sem margens @param x coordenada horizontal em pontos canto superior esquerdo @param y coordenada vertical em pontos canto superior esquerdo @param fontsize tamanho da fonte do texto a ser impresso @param foreColor forecolor do texto a ser impresso @param backgroundColor cor do retângulo preenchido @return void * / void drawBoxedString (const uint16_t x, const uint16_t y, const char * string, const uint16_t fontsize, const uint16_t foreColor, const uint16_t backgroundColor) {tft.setTextSize (fontsize); int16_t x1, y1; uint16_t w, h; tft.getTextBounds (string, x, y, &x1, &y1, &w, &h); tft.fillRect (x, y, w, h, backgroundColor); tft.setCursor (x, y); tft.setTextColor (foreColor); tft.print (string);} / ** Limpar a tela para os planos de fundo padrão @param void @return void * / void clearDialog (gameSize_type gameSize) {tft.fillRect (gameSize.x, gameSize.y, gameSize.width, gameSize .height, backgroundColor); tft.fillRect (gameSize.x, gameSize.y, gameSize.width, SCORE_SIZE, PRIMARY_DARK_COLOR);} ////////////////////////////// //////////////////////////////////////// READ UI SELECTION ///////////// //////////////////////////////////////////////////////// // * Verifica se o usuário está selecionando algum dos elementos da interface do usuário habilitados visíveis O retorno de chamada onTap do elemento selecionado é chamado e definido como pressionado @param lastSelecionado a última seleção @return the new selection * / int readUiSelection (game_type * game, game_state_type * state, const int16_t lastSelected) {int16_t xpos, ypos; // coordenadas da tela TSPoint tp =ts.getPoint (); //tp.x, tp.y são valores ADC // se estiver compartilhando pinos, você precisará corrigir as direções dos pinos da tela de toque pinMode (XM, OUTPUT); pinMode (YP, OUTPUT); // temos alguma pressão mínima que consideramos 'válida' // pressão de 0 significa sem pressão! if (tp.z> MINPRESSURE &&tp.z tft.width () / 2) {estado-> playerx + =2; } else {estado-> playerx - =2; } if (estado-> playerx> =tft.width () - jogo-> playerwidth) estado-> playerx =tft.width () - jogo-> playerwidth; if (estado-> playerx <0) estado-> playerx =0; return 1; }#ifdef DEMO_MODE state->playerx =(state->ballx>> game->exponent) - game->playerwidth / 2; if (state->playerx>=tft.width() - game->playerwidth) state->playerx =tft.width() - game->playerwidth; if (state->playerx <0) state->playerx =0;#endif return -1;}int waitForTouch() { int16_t xpos, ypos; //screen coordinates TSPoint tp =ts.getPoint(); //tp.x, tp.y are ADC values // if sharing pins, you'll need to fix the directions of the touchscreen pins pinMode(XM, OUTPUT); pinMode(YP, OUTPUT); // we have some minimum pressure we consider 'valid' // pressure of 0 means no pressing! if (tp.z> MINPRESSURE &&tp.z
Esquemas
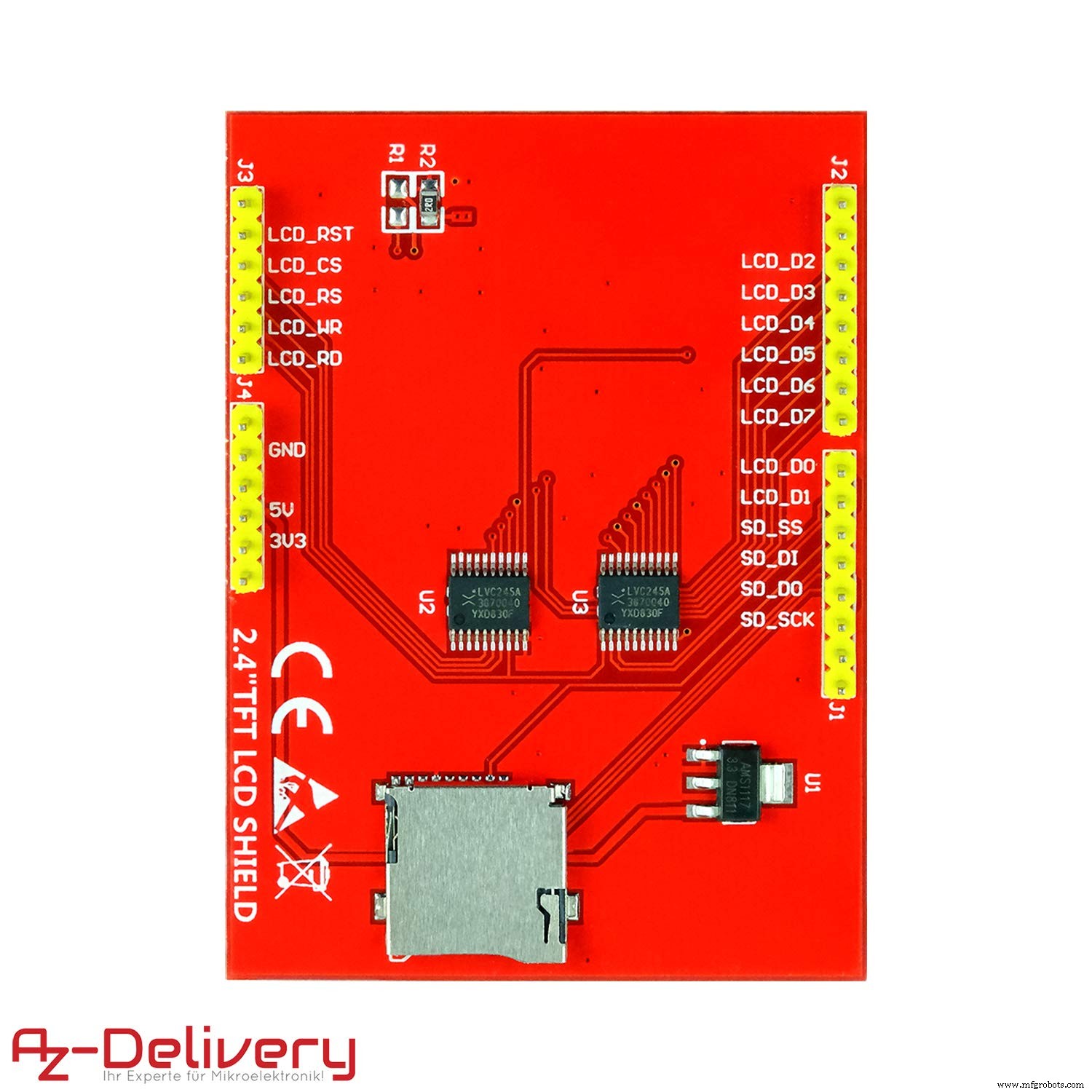
Processo de manufatura
- Jogo Arduino Gyroscope com MPU-6050
- Jogo Arduino Pong - Tela OLED
- Piano portátil de toque capacitivo
- Controlador de jogo Arduino
- Jogo Arduino Pong em Matriz 24x16 com MAX7219
- Jogo de Operação Gigante Animatronics Lego Minfig
- Adaptadores MIDI USB-BLE sem fio
- Jogo Pixel Chaser
- Reconhecimento de fala e síntese com Arduino
- Jogo Dino automatizado usando arduino